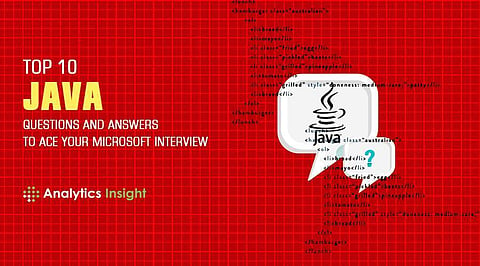
Aiming for a career at Microsoft is a worthy challenge given the company's excellent track record for technological innovation, generous compensation packages, and flexible work-life balance. Java questions for Microsoft interviews are mostly about data structures, algorithms, Oops concepts, etc. The interviewer never asks questions related to Java only. The interviewer can also ask questions from Arrays, LinkedLists, Stacks, Queues, Strings, Patterns, and Binary trees, etc. Are you looking for Java questions and answers for Microsoft interviews? You are at the right place; this article features the top 10 java questions and answers to ace your Microsoft interview.
In order to check whether the Binary Tree is BST or not, we simply check whether the left child node is smaller than the current node or not. We also check whether the right child node is greater than the current node or not. For a BST Binary tree, the left child should have smaller, and the right child should have greater value from its parent note. It is one of the crucial Java questions for Microsoft interview.
In order to find an element from an infinite sorted array, we set the index at position 100. If the element which we need to find is less from the index number, perform the binary search for the last 100 items. Else we set the index at position 200. We set the index by 100 until the item is greater. It is one of the top 10 java questions and answers to ace your Microsoft interview.
Each element of a linked list stores the address of its next element or node. In order to reverse a linked list, we need to store the address of the previous node in each node of the linked list. We can reverse the linked list by using recursion or by using iteration. We use the following steps to reverse a linked list:
We use two pointers, i.e., fast and slow at the time of iterating over the linked list. The slow and fast pointers move two and one nodes in each iteration, respectively. If the linked list contains a cycle, both pointers will meet at the same point during iteration. If both the pointers point to null, the linked list doesn't contain any loop or cycle in it.
Double-checked locking of Singleton is a way to ensure that only a single instance of a Singleton class is created through an application life cycle. The double-checked locking means that the code checks for an existing instance of Singleton class twice with and without locking to double ensure that no more than one instance of Singleton gets created. It is one of the top 10 java questions and answers to ace your Microsoft interview.
A transient variable is a special type of variable in Java that is initialized during de-serialization with its default value. At the time of Serialization, the value of the transient variable is not serialized. In order to prevent any object from being serialized, we use the transient variable. We can easily create a transient variable by using the transient keyword.
In Java, volatile is a keyword that is intended to address variable visibility problems. It is another way of making a class thread-safe. The thread-safe means that multiple threads can use a method or a class instance without any problem. It is one of the top 10 java questions and answers to ace your Microsoft interview.
We cannot override the private methods because we cannot access the private methods in the same way as non-private methods. The method overriding is possible in the child class only, and we cannot access the private methods in the child class. It is one of the crucial Java questions and answers for Microsoft interview.
The equals() and the hashcode() are two methods that we can override for an object to be used as the key in HashMap. It is one of the top 10 java questions and answers to ace your Microsoft interview.
Join our WhatsApp Channel to get the latest news, exclusives and videos on WhatsApp
_____________
Disclaimer: Analytics Insight does not provide financial advice or guidance. Also note that the cryptocurrencies mentioned/listed on the website could potentially be scams, i.e. designed to induce you to invest financial resources that may be lost forever and not be recoverable once investments are made. You are responsible for conducting your own research (DYOR) before making any investments. Read more here.