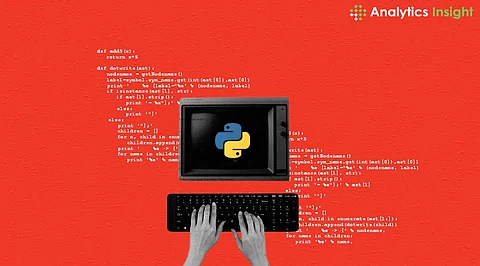
Python has become one of the most popular programming languages, known for its simplicity, versatility, and readability. Whether you're new to programming or coming from another language, mastering Python syntax is essential for building a solid foundation in Python programming. In this article, we will explore the top 10 essential Python syntaxes that every beginner should know to kickstart their journey into Python programming.
Variables are used to store data in Python and can include a variety of data types, including integers, floats, strings, lists, tuples, and dictionaries. Understanding how to declare variables and work with different data types is fundamental to writing Python code. Variables are created when you assign a value to them. They do not require explicit declaration, and their type is deduced from the assigned value.
x = 10
name = "Alice"
Comments are used to add notes or explanations within the code. They are ignored by the Python interpreter and help in documenting code making it more understandable to others. Comments are marked by # and are used to explain code.
# This is a single-line comment
The print () function is your gateway to displaying output in Python. It's simple and versatile, allowing you to print strings, numbers, or variables to the console.
print("Hello, World!")
The Python conditional operator, often known as the ternary operator, is a shorthand of writing an if-else statement. It is a way for evaluating a condition and returning one of two values based on the outcome of that condition. Conditional statements are used to run different blocks of code based on certain conditions. The if, elif, and else keywords are used to define conditional statements in Python.
if x > 0:
print("Positive number")
elif x == 0:
print("Zero")
else:
print("Negative number")
Python supports all basic arithmetic operations, including addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). These operators perform common mathematical operations on numeric values, such as integers and floats.
sum = 5 + 5 # 10
remainder = 10 % 3 # 1
Loops are used to iterate over a sequence of elements in Python. Loops, specifically for and while, let you execute a block of code multiple times. Python provides three ways to execute loops. While all the ways provide similar basic functionality, they differ in their syntax and condition-checking time.
# for loop
for i in range(5):
print(i)
# while loop
x = 0
while x < 5:
print(x)
x += 1
Functions are reusable code blocks that perform a specific task. They help you structure your code into logical units, making it more modular and maintainable. Functions are defined using the def keyword and are used to encapsulate reusable blocks of code.
Copy code
# Function definition
def greet(name):
print("Hello, " + name + "!")
# Function call
greet("John")
Strings are sequences of characters enclosed in single or double quotations. Python has various methods for manipulating strings, including concatenation, slicing, and formatting.
Copy code
# String concatenation
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
Error handling allows you to gracefully handle exceptions and prevent your program from crashing. The try and except keywords are used to catch and handle exceptions in Python code.
Copy code
try:
x = 10 / 0
except ZeroDivisionError:
print("Division by zero is not allowed")
Dictionaries are used to store key-value pairs in Python. They are mutable, unordered, and can store elements of different data types.
# Dictionary declaration
my_dict = {"name": "John", "age": 30, "city": "New York"}
Mastering Python syntax is crucial for beginners looking to become proficient in Python programming. Familiarizing yourself with these top ten important syntaxes will provide you with a solid understanding of Python fundamentals and prepare you to handle more advanced concepts and projects in Python programming. Whether you're building simple scripts, web applications, or machine learning models, these syntaxes will serve as the building blocks of your Python journey.
Join our WhatsApp Channel to get the latest news, exclusives and videos on WhatsApp
_____________
Disclaimer: Analytics Insight does not provide financial advice or guidance on cryptocurrencies and stocks. Also note that the cryptocurrencies mentioned/listed on the website could potentially be scams, i.e. designed to induce you to invest financial resources that may be lost forever and not be recoverable once investments are made. This article is provided for informational purposes and does not constitute investment advice. You are responsible for conducting your own research (DYOR) before making any investments. Read more here.