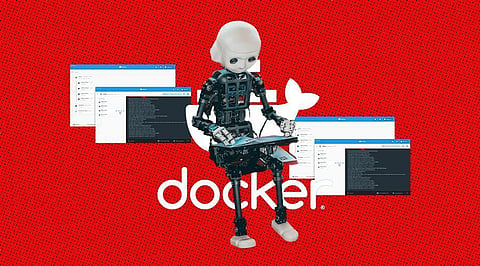
You can use your personal computer to run the ChatGPT locally using a docker desktop. You must first be familiar with the installation and configuration of the OpenAI API client before you can proceed. Then, try to figure out how to create a straightforward ChatGPT-like chatbot system.
Please note that this is the first part of this article where you will see how to get started with OpenAI and build a simple Pet Name Generator app. In the 2nd part of the article, you will see how to build a chatbot system. Let's get started.
To start, you must request an OpenAI API key. To create a secret key, go to the OpenAI API website.
Create the following example Node.js script to show how to run Chat GPT locally using the OpenAI API client:
const openai = require('openai');
// Set your OpenAI API key
openai.apiKey = "YOUR_API_KEY";
// Set the prompt for Chat GPT
const prompt = "What's your favorite color?";
// Set the model to use (in this case, Chat GPT)
const model = "chatbot";
// Generate a response from Chat GPT
openai.completions.create({
engine: model,
prompt: prompt,
max_tokens: 2048,
n: 1,
stop: '.',
temperature: 0.5,
}, (error, response) => {
console.log(response.choices[0].text);
});
Based on the supplied prompt, this script generates a response from ChatGPT using the openai.completions.create() method. The temperature and max tokens parameters can be used to regulate the response's length and originality, respectively.
Fork the Pet name-generating app source and try to build your version of a Dockerized solution to see how OpenAI operates. To start, you should try running the app directly from your local machine to make sure Docker wasn't needed.
git clone https://github.com/ajeetraina/openai-quickstart-node
cd openai-quickstart-node
npm install
cp .env.example .env
Add your API key to the newly created .env file
npm run build
You should now be able to access the app at http://localhost:3000!
Let us try to run the Pet Name Generator app in a Docker container. To do this, you will need to install Docker locally in your system. I recommend using Docker Desktop which is free of cost for personal usage.
Create a Dockerfile: In your project directory, create a file called Dockerfile and add the following content to it:
# Use the official Node.js 10 image as the base
FROM node:14
# Create a working directory
RUN mkdir -p /usr/src/app
# Set the working directory
WORKDIR /usr/src/app
# Copy the package.json and package-lock.json files
COPY package*.json /usr/src/app/
# Install the dependencies
RUN npm install
# Copy the rest of the application code
COPY . /usr/src/app
# Expose the application's port
EXPOSE 8080
# Run the application
CMD [ "npm", "run", "dev" ]
This Dockerfile specifies the base image (node:14) to use for the Docker container and installs the OpenAI API client. It also copies the app code to the container and sets the working directory to the app code.
docker build -t ajeetraina/chatbot-docker .
docker run -d -p 3000:3000 ajeetraina/chatbot-docker
Join our WhatsApp Channel to get the latest news, exclusives and videos on WhatsApp
_____________
Disclaimer: Analytics Insight does not provide financial advice or guidance. Also note that the cryptocurrencies mentioned/listed on the website could potentially be scams, i.e. designed to induce you to invest financial resources that may be lost forever and not be recoverable once investments are made. You are responsible for conducting your own research (DYOR) before making any investments. Read more here.