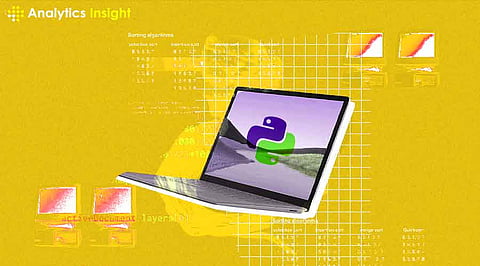
Data analysis is an integral part of modern data-driven decision-making, encompassing a broad array of techniques and tools to process, visualize, and interpret data. Python, a versatile programming language, has established itself as a staple in the data analysis landscape, primarily due to its powerful libraries: Pandas, NumPy, and Matplotlib. These libraries provide a robust framework for manipulating, analyzing, and visualizing data, making Python a preferred choice for data analysts and scientists.
Python's simplicity and readability, combined with its extensive libraries, make it an ideal language for data analysis. Among these libraries, Pandas, NumPy, and Matplotlib stand out due to their functionality and ease of use.
Pandas: This library offers data structures and functions designed to make data manipulation and analysis fast and straightforward. Its primary data structure, the DataFrame, is similar to a table in a database or an Excel spreadsheet.
NumPy: Short for Numerical Python, NumPy provides support for arrays, matrices, and a large collection of mathematical functions to efficiently operate on these data structures.
Matplotlib: This plotting library provides tools for creating static, animated, and interactive visualizations in Python, making it essential for data visualization.
NumPy, or Numerical Python, is the basic package for scientific computing using Python. It introduces support for arrays and matrices and a comprehensive collection of mathematical functions to operate on these data structures.
The core feature of NumPy is its array object, array. Unlike Python lists, NumPy arrays are designed for numerical operations and offer significant performance improvements due to their ability to handle large datasets efficiently through vectorized operations. Vectorization allows for the execution of operations on entire arrays rather than individual elements, thus optimizing computational performance.
NumPy provides a plethora of functions to perform mathematical operations on arrays, including trigonometric functions, statistical measures, and linear algebra operations. This makes it an invaluable tool for numerical analysis, enabling complex calculations and data transformations to be executed swiftly and accurately.
Pandas is built on top of NumPy and provides high-level data structures and functions designed to make data manipulation and analysis easy and intuitive. Pandas' fundamental data structures are series and dataframes.
A Pandas Series is a one-dimensional array-like object that can hold various data types and is capable of holding both integer and string labels, which makes it similar to a dictionary in Python. A data frame, on the other hand, is a two-dimensional table-like data structure with labeled axes (rows and columns). This makes it similar to a spreadsheet or an SQL table, which is particularly useful for handling heterogeneous data.
One of Pandas' key strengths is its ability to handle missing data. Functions like drop and filling allow for easy removal or imputation of missing values. Pandas also provides powerful tools for data transformation, such as merging, joining, and reshaping datasets, making it easier to prepare data for analysis.
Pandas excel at aggregating data based on various criteria using its group-by functionality. This is particularly useful for summarizing data and extracting meaningful insights. Aggregation functions such as mean, sum, and count can be applied to grouped data to derive statistical summaries, which are crucial in exploratory data analysis.
Matplotlib is the premier plotting library in Python, known for its versatility and extensive range of plotting options. It provides the foundation for creating static, animated, and interactive visualizations.
Matplotlib's Pyplot module offers a simple interface for creating basic plots such as line charts, bar charts, and scatter plots. These visualizations are essential for understanding data trends and distributions and facilitate better data interpretation.
Beyond basic plots, Matplotlib supports a wide array of advanced visualizations, including histograms, box plots, and heat maps. These plots help uncover more profound insights into the data, such as distribution patterns and correlations between variables.
Matplotlib is highly customizable, allowing users to tweak almost every aspect of a plot, from colors and line styles to labels and annotations. This flexibility is crucial for creating publication-quality visualizations that effectively communicate data insights.
One of the advantages of using Pandas and Matplotlib together is their seamless integration. Data stored in Pandas DataFrames can be easily plotted using Matplotlib, enabling a smooth workflow from data manipulation to visualization. This integration allows for efficient exploratory data analysis, where insights gleaned from visualizations can be immediately acted upon within the same coding environment.
By combining the capabilities of Pandas, NumPy, and Matplotlib, one can perform sophisticated data analysis tasks that go beyond basic manipulation and visualization.
Handling time series data is a common requirement in data analysis. Pandas offers robust support for time series, providing functions for resampling, shifting, and rolling window calculations. These tools are essential for analyzing trends, seasonality, and cyclic patterns in time-based data.
NumPy and Pandas offer extensive functionalities for statistical analysis, including descriptive statistics, probability distributions, and hypothesis testing. These statistical tools are crucial for validating data insights and making data-driven decisions.
Python’s data analysis libraries integrate well with machine learning libraries such as Scikit-learn. This integration allows for the seamless transition from data preprocessing and exploration to building and evaluating machine learning models. Data can be prepared and visualized using Pandas and Matplotlib, then fed into machine learning algorithms for predictive modeling.
To illustrate the power of these libraries, let’s consider a case study involving the Titanic dataset. This dataset contains information about the passengers aboard the Titanic, including whether they survived, their age, and their class.
The first step in analyzing the Titanic dataset is loading the data into a Pandas data frame. Once loaded, the data can be explored using functions like head, description, and info to get an overview of the dataset and its structure.
The Titanic dataset contains missing values, which need to be addressed. Pandas provide functions to handle missing data effectively. For instance, missing ages can be filled with the mean age, and missing embarkation points can be filled with the most common port.
Data Visualization
Visualizations can help uncover patterns in the data. For example, plotting the survival rate by passenger class using a bar plot can reveal insights into the likelihood of survival based on class. Similarly, histograms of age distributions can show the age demographics of the passengers.
Statistical tests can be performed to understand the significance of various features. For instance, a chi-square test can determine whether there is a significant association between passenger class and survival status.
Finally, the cleaned and visualized data can be used to build a predictive model. Features such as age, sex, and passenger class can be fed into a machine-learning algorithm to predict survival. The model’s performance can be evaluated using metrics like accuracy, precision, and recall.
The combination of Pandas, NumPy, and Matplotlib provides a powerful toolkit for data analysis in Python. NumPy’s efficient numerical computations, Pandas’ intuitive data manipulation capabilities, and Matplotlib’s extensive visualization options collectively enable comprehensive data analysis workflows. These libraries not only facilitate basic data processing and visualization but also support advanced analysis techniques, making Python a dominant language in the field of data science.
In summary, leveraging the strengths of Pandas, NumPy, and Matplotlib can significantly enhance the data analysis process. These libraries allow data analysts and scientists to handle data more effectively, uncover insights through visualizations, and perform complex analyses with ease.
What are the applications of NumPy Pandas and Matplotlib in Python?
Pandas is a Python package for data analysis. It is based on two Python libraries: Matplotlib for data visualization and Numpy for mathematical computations. Panda functions as a wrapper for these libraries, letting you use numerous Matplotlib and NumPy techniques with less code.
How is Pandas used for data analytics in Python?
Pandas is an open-source Python library. According to its official website, it is a versatile and user-friendly Python-based data analysis and manipulation application. Pandas, as previously noted, is based on NumPy, a Python library for scientific computing and data processing.
What use does Matplotlib serve in Python?
Matplotlib is a popular Python plotting toolkit for creating high-quality visualizations and graphs. It provides a variety of tools for creating different graphs, making data analysis, exploration, and presentation easier.
Why are pandas ideal for data analysis?
One of Pandas' most outstanding abilities is their adaptability. It seamlessly manages a variety of data sources, including CSV files, Excel spreadsheets, SQL databases, and JSON data. Pandas brings all of your data together, making data analysis and manipulation a smooth and enjoyable experience.
Join our WhatsApp Channel to get the latest news, exclusives and videos on WhatsApp
_____________
Disclaimer: Analytics Insight does not provide financial advice or guidance. Also note that the cryptocurrencies mentioned/listed on the website could potentially be scams, i.e. designed to induce you to invest financial resources that may be lost forever and not be recoverable once investments are made. You are responsible for conducting your own research (DYOR) before making any investments. Read more here.